EditableMesh
Instance which allows for the runtime creation and manipulation of meshes.
Memory category | Instances |
---|
Member index 88
Removed member index 15
History | Member | |
---|---|---|
660 | CreateMeshPartAsync(initialSize: Vector3, options: Dictionary = nil): MeshPart | |
648 | GetAdjacentTriangles(triangleId: int64): Array | |
649 | GetContent(): Content | |
648 | GetTriangleVertices(triangleId: int64): Tuple | |
648 | GetTriangles(): Array | |
648 | GetVertexColor(vertexId: int64): Color3 | |
648 | GetVertexColorAlpha(vertexId: int64): float | |
648 | GetVertexNormal(vertexId: int64): Vector3 | |
604 | Raycast(origin: Vector3, direction: Vector3): Tuple | |
648 | RemoveTriangle(triangleId: int64): null | |
648 | RemoveVertex(vertexId: int64): null | |
648 | SetVertexColor(vertexId: int64, color: Color3): null | |
648 | SetVertexColorAlpha(vertexId: int64, alpha: float): null | |
648 | SetVertexNormal(vertexId: int64, vnormal: Vector3): null |
Description
EditableMesh
changes the applied visual mesh when linked to a
MeshPart, allowing for querying and modification of the mesh both in
Studio and in experience.
An EditableMesh
can be created from an existing Content of a
MeshPart or a mesh ID using
AssetService:CreateEditableMeshAsync(), or a blank EditableMesh
can
be created with AssetService:CreateEditableMesh(). It can then be
displayed, modified, and its collision model updated. Not all of the steps are
necessary; for example, you might want to create an EditableMesh
just to
raycast without ever displaying it.
An EditableMesh
is displayed when it's linked to a new MeshPart,
through AssetService:CreateMeshPartAsync(). You can create more
MeshPart instances that reference the same EditableMesh
Content, or link to an existing MeshPart through
MeshPart:ApplyMesh().
To recalculate collision and fluid geometry after editing, you can again call AssetService:CreateMeshPartAsync() and MeshPart:ApplyMesh() to update an existing MeshPart. It's generally recommended to do this at the end of a conceptual edit, not after individual calls to methods that manipulate geometry. Visual changes to the mesh will always be immediately reflected by the engine, without the need to call AssetService:CreateMeshPartAsync().
Enabling EditableMesh for Published Experiences
For security purposes, using EditableMesh
fails by default for published
experiences. To enable usage, you must be 13+ age verified and ID verified.
After you are verified, open Studio's
Game Settings, select Security, and
enable the Allow Mesh / Image APIs toggle.
Permissions
To prevent misuse, AssetService:CreateEditableMeshAsync() will only allow you to load and edit mesh assets:
- That are owned by the creator of the experience (if the experience is owned by an individual).
- That are owned by a group (if the experience is owned by the group).
- That are owned by the logged in Studio user (if the place file has not yet been saved or published to Roblox).
The APIs throw an error if they are used to load an asset that does not meet the criteria above.
Fixed-Size Meshes
When creating an EditableMesh
from an existing mesh asset (via
AssetService:CreateEditableMeshAsync()), the resulting editable mesh
is fixed-size by default. Fixed-size meshes are more efficient in terms of
memory but you cannot change the number of vertices, faces, or attributes.
Only the values of vertex attributes and positions can be edited.
Stable Vertex/Face IDs
Many EditableMesh
methods take vertex, normal, UV, color and
face IDs. These are represented as integers in Luau but they require some
special handling. The main difference is that IDs are stable and they remain
the same even if other parts of the mesh change. For example, if an
EditableMesh
has five vertices {1, 2, 3, 4, 5}
and you remove vertex 4
,
the new vertices will be {1, 2, 3, 5}
.
Note that the IDs are not guaranteed to be in order and there may be holes in the numbering, so when iterating through vertices or faces, you should iterate through the table returned by GetVertices() or GetFaces().
Split Vertex Attributes
A vertex is a corner of a face, and topologically connects faces together. Vertices can have several attributes: position, normal, UV coordinate, color, and transparency.
Sometimes it's useful for all faces that touch a vertex to use the same attribute values, but sometimes you'll want different faces to use different attribute values on the same vertex. For example, on a smooth sphere, each vertex will only have a single normal. In contrast, at the corner of a cube, the vertex will have 3 different normals (one for each adjacent face). You can also have seams in the UV coordinates or sharp changes in the vertex colors.
When creating faces, every vertex will by default have one of each attribute: one normal, one UV coordinate, and one color/transparency. If you want to create a seam, you should create new attributes and set them on the face. For example, this code will create a sharp cube:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
|
Winding
Mesh faces have a front side and a back side. When drawing meshes, only the
front of the faces are drawn by default, although you can change this by
setting the mesh' DoubleSided property to true
.
The order of the vertices around the face determines whether you are looking at the front or the back. The front of the face is visible when the vertices go counterclockwise around it.
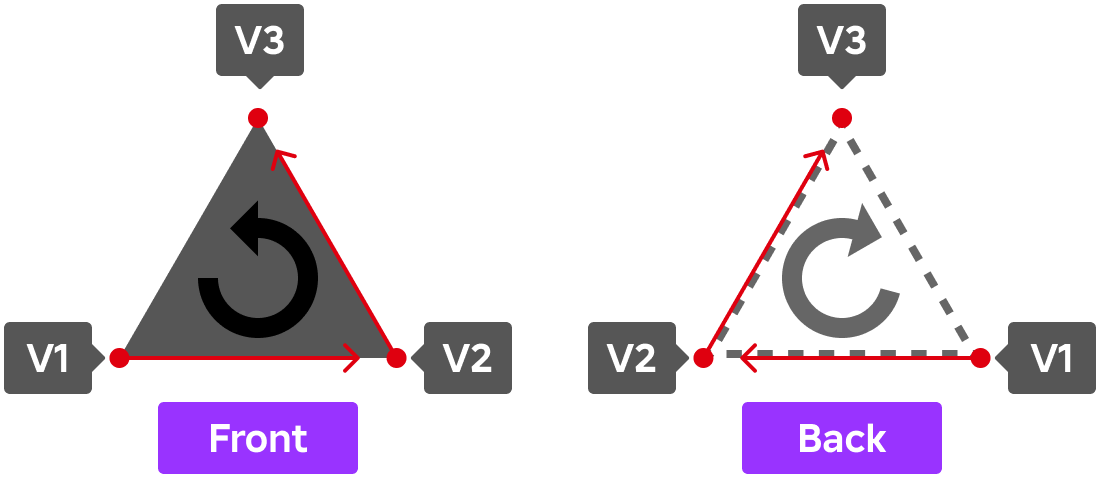
FACS Poses
Animatable heads use the Facial Action Coding System (FACS). See the FACS poses reference for helpful information when using GetFacsPoses() and similar methods.
Each FACS pose is specified by an FacsActionUnit value. For the FACS pose, virtual bones can each have a CFrame that transforms the bones' initial CFrame in the bind pose of the mesh into the CFrame for that FACS action unit's pose. All bone CFrames are in the mesh's local space.
These FACS poses are blended together during animation. Sometimes, the blending of the base poses produces poor results. In those cases, you can override the blending of specific combinations of base poses with a corrective pose that is more pleasing. A corrective pose is specified by 2 or 3 FacsActionUnit values. Like a base FACS pose, for a corrective pose, virtual bones can each have a CFrame that transforms the bones' initial CFrame in the bind pose of the mesh into the CFrame for that FACS corrective.
Limitations
EditableMesh
currently has a limit of 60,000 vertices and 20,000 triangles.
Attempting to add too many vertices or triangles will cause an error.
History 224
- 672 Change Tags of SkinningEnabled from [] to [Deprecated]
- 670 Add SetVertexBones
- 670 Add SetVertexBoneWeights
- 670 Add SetFacsPose
- 670 Add SetFacsCorrectivePose
- 670 Add SetFacsBonePose
- 670 Add SetBoneParent
- 670 Add SetBoneName
- 670 Add SetBoneIsVirtual
- 670 Add SetBoneCFrame
- 670 Add RemoveBone
- 670 Add GetVertexBones
- 670 Add GetVertexBoneWeights
- 670 Add GetFacsPoses
- 670 Add GetFacsPose
- 670 Add GetFacsCorrectivePoses
- 670 Add GetFacsCorrectivePose
- 670 Add GetBones
- 670 Add GetBoneParent
- 670 Add GetBoneName
- 670 Add GetBoneIsVirtual
- 670 Add GetBoneCFrame
- 670 Add GetBoneByName
- 670 Add AddBone
- 667 Add SetVertexFaceUV
- 667 Add SetVertexFaceNormal
- 667 Add SetVertexFaceColor
- 667 Add GetVerticesWithUV
- 667 Add GetVerticesWithNormal
- 667 Add GetVerticesWithColor
- 667 Change Tags of GetVerticesWithAttribute from [] to [Deprecated]
- 667 Add GetVertexUVs
- 667 Add GetVertexNormals
- 667 Add GetVertexFaces
- 667 Add GetVertexFaceUV
- 667 Add GetVertexFaceNormal
- 667 Add GetVertexFaceColor
- 667 Add GetVertexColors
- 667 Add GetFacesWithUV
- 667 Add GetFacesWithNormal
- 667 Add GetFacesWithColor
- 667 Change Tags of GetFacesWithAttribute from [] to [Deprecated]
- 660 Remove CreateMeshPartAsync
- 650 Add GetSize
- 650 Add GetCenter
- 649 Remove GetContent
- 649 Add Destroy
- 649 Change Tags of CreateMeshPartAsync from [Yields] to [Yields, Deprecated]
- 649 Change PreferredDescriptor of CreateMeshPartAsync from to CreateMeshPartAsync
- 649 Change CanSave of from false to true
- 649 Change CanLoad of from false to true
- 649 Change Tags of EditableMesh from [] to [NotCreatable]
- 648 Remove SetVertexNormal
- 648 Remove SetVertexColorAlpha
- 648 Remove SetVertexColor
- 648 Remove RemoveVertex
- 648 Remove RemoveTriangle
- 648 Remove GetVertexNormal
- 648 Remove GetVertexColorAlpha
- 648 Remove GetVertexColor
- 648 Remove GetTriangles
- 648 Remove GetTriangleVertices
- 648 Add GetContent
- 648 Remove GetAdjacentTriangles
- 648 Change Parameters of CreateMeshPartAsync from (options: Dictionary = nil) to (initialSize: Vector3, options: Dictionary = nil)
- 648 Change Default of SkinningEnabled from false to __api_dump_failed_to_create_class__
- 648 Change Default of from false to __api_dump_failed_to_create_class__
- 648 Add FixedSize
- 648 Change Superclass of EditableMesh from DataModelMesh to Object
- 644 Change Tags of SetFaceVertices from [] to [CustomLuaState]
- 644 Change Tags of SetFaceUVs from [] to [CustomLuaState]
- 644 Change Tags of SetFaceNormals from [] to [CustomLuaState]
- 644 Change Tags of SetFaceColors from [] to [CustomLuaState]
- 644 Change Tags of GetUVs from [] to [CustomLuaState]
- 644 Change Tags of GetNormals from [] to [CustomLuaState]
- 644 Change Tags of GetFaces from [] to [CustomLuaState]
- 644 Change Tags of GetFaceVertices from [] to [CustomLuaState]
- 644 Change Tags of GetFaceUVs from [] to [CustomLuaState]
- 644 Change Tags of GetFaceNormals from [] to [CustomLuaState]
- 644 Change Tags of GetFaceColors from [] to [CustomLuaState]
- 644 Change Tags of GetColors from [] to [CustomLuaState]
- 640 Add Triangulate
- 640 Change Tags of SetVertexNormal from [] to [Deprecated]
- 640 Change Tags of SetVertexColorAlpha from [] to [Deprecated]
- 640 Change Tags of SetVertexColor from [] to [Deprecated]
- 640 Change Parameters of SetUV from (vertexId: int64, uv: Vector2) to (uvId: int64, uv: Vector2)
- 640 Add SetNormal
- 640 Add SetFaceVertices
- 640 Add SetFaceUVs
- 640 Add SetFaceNormals
- 640 Add SetFaceColors
- 640 Add SetColorAlpha
- 640 Add SetColor
- 640 Add ResetNormal
- 640 Change Tags of RemoveVertex from [] to [Deprecated]
- 640 Add RemoveUnused
- 640 Change Tags of RemoveTriangle from [] to [Deprecated]
- 640 Add RemoveFace
- 640 Add MergeVertices
- 640 Add IdDebugString
- 640 Add GetVerticesWithAttribute
- 640 Change Tags of GetVertexNormal from [] to [Deprecated]
- 640 Change Tags of GetVertexColorAlpha from [] to [Deprecated]
- 640 Change Tags of GetVertexColor from [] to [Deprecated]
- 640 Add GetUVs
- 640 Change Parameters of GetUV from (vertexId: int64) to (uvId: int64)
- 640 Change ReturnType of GetUV from Vector2 to Vector2?
- 640 Change Tags of GetTriangles from [] to [Deprecated]
- 640 Change Tags of GetTriangleVertices from [] to [Deprecated]
- 640 Add GetNormals
- 640 Add GetNormal
- 640 Add GetFacesWithAttribute
- 640 Add GetFaces
- 640 Add GetFaceVertices
- 640 Add GetFaceUVs
- 640 Add GetFaceNormals
- 640 Add GetFaceColors
- 640 Add GetColors
- 640 Add GetColorAlpha
- 640 Add GetColor
- 640 Change Tags of GetAdjacentTriangles from [] to [Deprecated]
- 640 Add GetAdjacentFaces
- 640 Add AddUV
- 640 Add AddNormal
- 640 Add AddColor
- 636 Add
- 630 Change Tags of from [Hidden, NotReplicated, NotScriptable] to [Hidden, NotScriptable]
- 630 Change Tags of EditableMesh from [NotReplicated] to []
- 624 Add SkinningEnabled
- 619 Change Parameters of CreateMeshPartAsync from (collisionFidelity: CollisionFidelity) to (options: Dictionary = nil)
- 607 Remove
- 604 Change Parameters of SetVertexNormal from (vertexId: int, vnormal: Vector3) to (vertexId: int64, vnormal: Vector3)
- 604 Change Parameters of SetVertexColorAlpha from (vertexId: int, alpha: float) to (vertexId: int64, alpha: float)
- 604 Change Parameters of SetVertexColor from (vertexId: int, color: Color3) to (vertexId: int64, color: Color3)
- 604 Change Parameters of SetUV from (vertexId: int, uv: Vector2) to (vertexId: int64, uv: Vector2)
- 604 Change Parameters of SetPosition from (vertexId: int, p: Vector3) to (vertexId: int64, p: Vector3)
- 604 Change Parameters of RemoveVertex from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of RemoveTriangle from (triangleId: int) to (triangleId: int64)
- 604 Add RaycastLocal
- 604 Remove Raycast
- 604 Change Parameters of GetVertexNormal from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetVertexColorAlpha from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetVertexColor from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetUV from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetTriangleVertices from (triangleId: int) to (triangleId: int64)
- 604 Change Parameters of GetPosition from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetAdjacentVertices from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetAdjacentTriangles from (triangleId: int) to (triangleId: int64)
- 604 Change ReturnType of FindClosestVertex from int to int64
- 604 Change ReturnType of AddVertex from int to int64
- 604 Change ReturnType of AddTriangle from int to int64
- 604 Change Parameters of AddTriangle from (vertexId0: int, vertexId1: int, vertexId2: int) to (vertexId0: int64, vertexId1: int64, vertexId2: int64)
- 604 Change Tags of EditableMesh from [] to [NotReplicated]
- 603 Change Parameters of SetVertexNormal from (vertexId: int64, vnormal: Vector3) to (vertexId: int, vnormal: Vector3)
- 603 Change Parameters of SetVertexColorAlpha from (vertexId: int64, alpha: float) to (vertexId: int, alpha: float)
- 603 Change Parameters of SetVertexColor from (vertexId: int64, color: Color3) to (vertexId: int, color: Color3)
- 603 Change Parameters of SetUV from (vertexId: int64, uv: Vector2) to (vertexId: int, uv: Vector2)
- 603 Change Parameters of SetPosition from (vertexId: int64, p: Vector3) to (vertexId: int, p: Vector3)
- 603 Change Parameters of RemoveVertex from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of RemoveTriangle from (triangleId: int64) to (triangleId: int)
- 603 Remove RaycastLocal
- 603 Add Raycast
- 603 Change Parameters of GetVertexNormal from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetVertexColorAlpha from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetVertexColor from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetUV from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetTriangleVertices from (triangleId: int64) to (triangleId: int)
- 603 Change Parameters of GetPosition from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetAdjacentVertices from (vertexId: int64) to (vertexId: int)
- 603 Change Parameters of GetAdjacentTriangles from (triangleId: int64) to (triangleId: int)
- 603 Change ReturnType of FindClosestVertex from int64 to int
- 603 Change ReturnType of AddVertex from int64 to int
- 603 Change ReturnType of AddTriangle from int64 to int
- 603 Change Parameters of AddTriangle from (vertexId0: int64, vertexId1: int64, vertexId2: int64) to (vertexId0: int, vertexId1: int, vertexId2: int)
- 603 Change Tags of EditableMesh from [NotReplicated] to []
- 604 Change Parameters of SetVertexNormal from (vertexId: int, vnormal: Vector3) to (vertexId: int64, vnormal: Vector3)
- 604 Change Parameters of SetVertexColorAlpha from (vertexId: int, alpha: float) to (vertexId: int64, alpha: float)
- 604 Change Parameters of SetVertexColor from (vertexId: int, color: Color3) to (vertexId: int64, color: Color3)
- 604 Change Parameters of SetUV from (vertexId: int, uv: Vector2) to (vertexId: int64, uv: Vector2)
- 604 Change Parameters of SetPosition from (vertexId: int, p: Vector3) to (vertexId: int64, p: Vector3)
- 604 Change Parameters of RemoveVertex from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of RemoveTriangle from (triangleId: int) to (triangleId: int64)
- 604 Add RaycastLocal
- 604 Remove Raycast
- 604 Change Parameters of GetVertexNormal from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetVertexColorAlpha from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetVertexColor from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetUV from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetTriangleVertices from (triangleId: int) to (triangleId: int64)
- 604 Change Parameters of GetPosition from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetAdjacentVertices from (vertexId: int) to (vertexId: int64)
- 604 Change Parameters of GetAdjacentTriangles from (triangleId: int) to (triangleId: int64)
- 604 Change ReturnType of FindClosestVertex from int to int64
- 604 Change ReturnType of AddVertex from int to int64
- 604 Change ReturnType of AddTriangle from int to int64
- 604 Change Parameters of AddTriangle from (vertexId0: int, vertexId1: int, vertexId2: int) to (vertexId0: int64, vertexId1: int64, vertexId2: int64)
- 604 Change Tags of EditableMesh from [] to [NotReplicated]
- 602 Add SetVertexNormal
- 602 Add SetVertexColorAlpha
- 602 Add SetVertexColor
- 602 Add SetUV
- 602 Add SetPosition
- 602 Add RemoveVertex
- 602 Add RemoveTriangle
- 602 Add Raycast
- 602 Add GetVertices
- 602 Add GetVertexNormal
- 602 Add GetVertexColorAlpha
- 602 Add GetVertexColor
- 602 Add GetUV
- 602 Add GetTriangles
- 602 Add GetTriangleVertices
- 602 Add GetPosition
- 602 Add GetAdjacentVertices
- 602 Add GetAdjacentTriangles
- 602 Add FindVerticesWithinSphere
- 602 Add FindClosestVertex
- 602 Add FindClosestPointOnSurface
- 602 Add CreateMeshPartAsync
- 602 Add AddVertex
- 602 Add AddTriangle
- 602 Add
- 602 Add
- 602 Add EditableMesh
Members 88
AddBone
Parameters (1) | ||
---|---|---|
boneProperties | Dictionary | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
AddColor
Parameters (2) | ||
---|---|---|
color | Color3 | |
alpha | float | |
Returns (1) | ||
int64 |
Adds a new color to the geometry and returns a stable color ID.
Thread safety | Unsafe |
---|
AddNormal
Parameters (1) | ||
---|---|---|
normal | Vector3? | |
Returns (1) | ||
int64 |
Adds a new normal to the geometry and returns a stable normal ID. If the normal value isn't specified, the normal will be automatically calculated.
Thread safety | Unsafe |
---|
AddTriangle
Parameters (3) | ||
---|---|---|
vertexId0 | int64 | |
vertexId1 | int64 | |
vertexId2 | int64 | |
Returns (1) | ||
int64 |
Adds a new triangle to the mesh and returns a stable face ID.
Thread safety | Unsafe |
---|
History 7
- 604 Change ReturnType of AddTriangle from int to int64
- 604 Change Parameters of AddTriangle from (vertexId0: int, vertexId1: int, vertexId2: int) to (vertexId0: int64, vertexId1: int64, vertexId2: int64)
- 603 Change ReturnType of AddTriangle from int64 to int
- 603 Change Parameters of AddTriangle from (vertexId0: int64, vertexId1: int64, vertexId2: int64) to (vertexId0: int, vertexId1: int, vertexId2: int)
- 604 Change ReturnType of AddTriangle from int to int64
- 604 Change Parameters of AddTriangle from (vertexId0: int, vertexId1: int, vertexId2: int) to (vertexId0: int64, vertexId1: int64, vertexId2: int64)
- 602 Add AddTriangle
AddUV
Parameters (1) | ||
---|---|---|
uv | Vector2 | |
Returns (1) | ||
int64 |
Adds a new UV to the geometry and returns a stable UV ID.
Thread safety | Unsafe |
---|
AddVertex
Parameters (1) | ||
---|---|---|
p | Vector3 | |
Returns (1) | ||
int64 |
Adds a new vertex to the geometry and returns a stable vertex ID.
Thread safety | Unsafe |
---|
Destroy
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
null |
Destroys the contents of the mesh, immediately reclaiming used memory.
Thread safety | Unsafe |
---|
FindClosestPointOnSurface
Parameters (1) | ||
---|---|---|
point | Vector3 | |
Returns (1) | ||
Tuple |
Finds the closest point on the mesh's surface. Returns the face ID, point on the mesh in local object space, and the barycentric coordinate of the position within the face. See RaycastLocal() for more information on barycentric coordinates.
Thread safety | Unsafe |
---|
History 1
FindClosestVertex
Parameters (1) | ||
---|---|---|
toThisPoint | Vector3 | |
Returns (1) | ||
int64 |
Finds the closest vertex to a specific point in space and returns a stable vertex ID.
Thread safety | Unsafe |
---|
History 4
- 604 Change ReturnType of FindClosestVertex from int to int64
- 603 Change ReturnType of FindClosestVertex from int64 to int
- 604 Change ReturnType of FindClosestVertex from int to int64
- 602 Add FindClosestVertex
FindVerticesWithinSphere
Parameters (2) | ||
---|---|---|
center | Vector3 | |
radius | float | |
Returns (1) | ||
Array |
Finds all vertices within a specific sphere and returns a list of stable vertex IDs.
Thread safety | Unsafe |
---|
History 1
FixedSize
Type | Default | |
---|---|---|
bool | __api_dump_failed_to_create_class__ |
Fixed-sized meshes allow changing the values of vertex attributes but do not allow vertices and triangles to be added or deleted.
Write security | RobloxSecurity |
---|---|
Thread safety | ReadSafe |
Category | Data |
Loaded/Saved | false/true |
GetAdjacentFaces
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
Array |
Given a stable face ID, returns a list of adjacent faces.
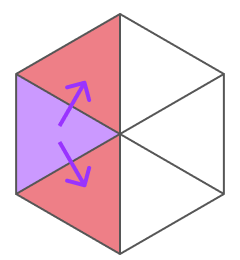
Thread safety | Unsafe |
---|
History 1
- 640 Add GetAdjacentFaces
GetAdjacentVertices
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Given a stable vertex ID, returns a list of adjacent vertices.
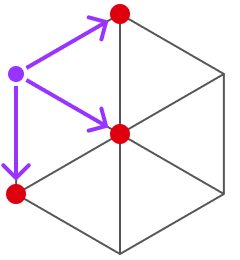
Thread safety | Unsafe |
---|
History 4
- 604 Change Parameters of GetAdjacentVertices from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetAdjacentVertices from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetAdjacentVertices from (vertexId: int) to (vertexId: int64)
- 602 Add GetAdjacentVertices
GetBoneByName
Parameters (1) | ||
---|---|---|
boneName | string | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetBoneByName
GetBoneCFrame
Parameters (1) | ||
---|---|---|
boneId | int64 | |
Returns (1) | ||
CFrame |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetBoneCFrame
GetBoneIsVirtual
Parameters (1) | ||
---|---|---|
boneId | int64 | |
Returns (1) | ||
bool |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetBoneIsVirtual
GetBoneName
Parameters (1) | ||
---|---|---|
boneId | int64 | |
Returns (1) | ||
string |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetBoneName
GetBoneParent
Parameters (1) | ||
---|---|---|
boneId | int64 | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetBoneParent
GetBones
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
GetCenter
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Vector3 |
Thread safety | Unsafe |
---|
GetColor
Parameters (1) | ||
---|---|---|
colorId | int64 | |
Returns (1) | ||
Color3? |
Returns the color for the given color ID.
Thread safety | Unsafe |
---|
GetColorAlpha
Parameters (1) | ||
---|---|---|
colorId | int64 | |
Returns (1) | ||
float? |
Returns the color alpha (transparency) at the given stable color ID.
Thread safety | Unsafe |
---|
History 1
- 640 Add GetColorAlpha
GetColors
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Returns all colors of the mesh as a list of stable color IDs.
Thread safety | Unsafe |
---|
GetFaceColors
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
Array |
Returns the face's color IDs for the vertices on the face.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of GetFaceColors from [] to [CustomLuaState]
- 640 Add GetFaceColors
GetFaceNormals
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
Array |
Returns the face's normal IDs for the vertices on the face.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of GetFaceNormals from [] to [CustomLuaState]
- 640 Add GetFaceNormals
GetFaceUVs
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
Array |
Returns the face's UV IDs for the vertices on the face.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of GetFaceUVs from [] to [CustomLuaState]
- 640 Add GetFaceUVs
GetFaceVertices
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
Array |
Returns the face's vertex IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of GetFaceVertices from [] to [CustomLuaState]
- 640 Add GetFaceVertices
GetFaces
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Returns all faces of the mesh as a list of stable face IDs.
Thread safety | Unsafe |
---|
GetFacesWithAttribute
Parameters (1) | ||
---|---|---|
id | int64 | |
Returns (1) | ||
Array |
Returns a list of faces that use a given vertex ID, normal ID, UV ID, or color ID.
Thread safety | Unsafe |
---|
History 2
- 667 Change Tags of GetFacesWithAttribute from [] to [Deprecated]
- 640 Add GetFacesWithAttribute
GetFacesWithColor
Parameters (1) | ||
---|---|---|
colorId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetFacesWithColor
GetFacesWithNormal
Parameters (1) | ||
---|---|---|
normalId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
GetFacesWithUV
Parameters (1) | ||
---|---|---|
uvId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetFacesWithUV
GetFacsCorrectivePose
Parameters (1) | ||
---|---|---|
actions | Array | |
Returns (1) | ||
Tuple |
Thread safety | Unsafe |
---|
History 1
GetFacsCorrectivePoses
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
GetFacsPose
Parameters (1) | ||
---|---|---|
action | FacsActionUnit | |
Returns (1) | ||
Tuple |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetFacsPose
GetFacsPoses
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetFacsPoses
GetNormal
Parameters (1) | ||
---|---|---|
normalId | int64 | |
Returns (1) | ||
Vector3? |
Returns the normal vector for the given normal ID.
Thread safety | Unsafe |
---|
GetNormals
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Returns all normals of the mesh as a list of stable normal IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of GetNormals from [] to [CustomLuaState]
- 640 Add GetNormals
GetPosition
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Vector3 |
Gets the position of a vertex in the mesh's local object space.
Thread safety | Unsafe |
---|
History 4
- 604 Change Parameters of GetPosition from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetPosition from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetPosition from (vertexId: int) to (vertexId: int64)
- 602 Add GetPosition
GetSize
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Vector3 |
Thread safety | Unsafe |
---|
GetUV
Parameters (1) | ||
---|---|---|
uvId | int64 | |
Returns (1) | ||
Vector2? |
Returns UV coordinates at the given UV ID.
Thread safety | Unsafe |
---|
History 6
- 640 Change Parameters of GetUV from (vertexId: int64) to (uvId: int64)
- 640 Change ReturnType of GetUV from Vector2 to Vector2?
- 604 Change Parameters of GetUV from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetUV from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetUV from (vertexId: int) to (vertexId: int64)
- 602 Add GetUV
GetUVs
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Returns all UVs of the mesh as a list of stable UV IDs.
Thread safety | Unsafe |
---|
GetVertexBoneWeights
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
GetVertexBones
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 670 Add GetVertexBones
GetVertexColors
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVertexColors
GetVertexFaceColor
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
History 1
GetVertexFaceNormal
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
History 1
GetVertexFaceUV
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
Returns (1) | ||
int64 |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVertexFaceUV
GetVertexFaces
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVertexFaces
GetVertexNormals
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVertexNormals
GetVertexUVs
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVertexUVs
GetVertices
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Returns all vertices as a list of stable vertex IDs.
Thread safety | Unsafe |
---|
History 1
- 602 Add GetVertices
GetVerticesWithAttribute
Parameters (1) | ||
---|---|---|
id | int64 | |
Returns (1) | ||
Array |
Returns a list of vertices that use a given face ID, normal ID, UV ID, or color ID.
Thread safety | Unsafe |
---|
History 2
- 667 Change Tags of GetVerticesWithAttribute from [] to [Deprecated]
- 640 Add GetVerticesWithAttribute
GetVerticesWithColor
Parameters (1) | ||
---|---|---|
colorId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
GetVerticesWithNormal
Parameters (1) | ||
---|---|---|
normalId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
GetVerticesWithUV
Parameters (1) | ||
---|---|---|
uvId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 1
- 667 Add GetVerticesWithUV
IdDebugString
Parameters (1) | ||
---|---|---|
id | int64 | |
Returns (1) | ||
string |
Returns a string describing a stable ID, useful for debugging purposes,
like f17
or v12
, containing the type, ID number, and version.
Thread safety | Unsafe |
---|
History 1
- 640 Add IdDebugString
MergeVertices
Parameters (1) | ||
---|---|---|
mergeTolerance | float | |
Returns (1) | ||
Map |
Merges vertices that touch together, to use a single vertex ID but keep the other original attribute IDs.
Thread safety | Unsafe |
---|
History 1
- 640 Add MergeVertices
RaycastLocal
Parameters (2) | ||
---|---|---|
origin | Vector3 | |
direction | Vector3 | |
Returns (1) | ||
Tuple |
Casts a ray and returns a point of intersection, face ID, and barycentric coordinates. The inputs and outputs of this method are in the mesh's local object space.
A barycentric coordinate is a way of specifying a point within a face as a weighted combination of the 3 vertices of the face. This is useful as a general way of blending vertex attributes. See this method's code sample as an illustration.
Thread safety | Unsafe |
---|
History 3
- 604 Add RaycastLocal
- 603 Remove RaycastLocal
- 604 Add RaycastLocal
RemoveBone
Parameters (1) | ||
---|---|---|
boneId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add RemoveBone
RemoveFace
Parameters (1) | ||
---|---|---|
faceId | int64 | |
Returns (1) | ||
null |
Removes a face using its stable face ID.
Thread safety | Unsafe |
---|
History 1
- 640 Add RemoveFace
RemoveUnused
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Removes all vertices, normals, UVs, and colors which are not used in any face, and returns the removed IDs.
Thread safety | Unsafe |
---|
History 1
- 640 Add RemoveUnused
ResetNormal
Parameters (1) | ||
---|---|---|
normalId | int64 | |
Returns (1) | ||
null |
Reset this normal ID to be automatically calculated based on the shape of the mesh, instead of manually set.
Thread safety | Unsafe |
---|
History 1
- 640 Add ResetNormal
SetBoneCFrame
Parameters (2) | ||
---|---|---|
boneId | int64 | |
cframe | CFrame | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetBoneCFrame
SetBoneIsVirtual
Parameters (2) | ||
---|---|---|
boneId | int64 | |
virtual | bool | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetBoneIsVirtual
SetBoneName
Parameters (2) | ||
---|---|---|
boneId | int64 | |
name | string | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetBoneName
SetBoneParent
Parameters (2) | ||
---|---|---|
boneId | int64 | |
parentBoneId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetBoneParent
SetColor
Parameters (2) | ||
---|---|---|
colorId | int64 | |
color | Color3 | |
Returns (1) | ||
null |
Sets the color for a color ID.
Thread safety | Unsafe |
---|
SetColorAlpha
Parameters (2) | ||
---|---|---|
colorId | int64 | |
alpha | float | |
Returns (1) | ||
null |
Sets the color alpha (transparency) for a color ID.
Thread safety | Unsafe |
---|
History 1
- 640 Add SetColorAlpha
SetFaceColors
Parameters (2) | ||
---|---|---|
faceId | int64 | |
ids | Array | |
Returns (1) | ||
null |
Sets the face's vertex colors to new color IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of SetFaceColors from [] to [CustomLuaState]
- 640 Add SetFaceColors
SetFaceNormals
Parameters (2) | ||
---|---|---|
faceId | int64 | |
ids | Array | |
Returns (1) | ||
null |
Sets the face's vertex normals to new normal IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of SetFaceNormals from [] to [CustomLuaState]
- 640 Add SetFaceNormals
SetFaceUVs
Parameters (2) | ||
---|---|---|
faceId | int64 | |
ids | Array | |
Returns (1) | ||
null |
Sets the face's vertex UVs to new UV IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of SetFaceUVs from [] to [CustomLuaState]
- 640 Add SetFaceUVs
SetFaceVertices
Parameters (2) | ||
---|---|---|
faceId | int64 | |
ids | Array | |
Returns (1) | ||
null |
Sets the face's vertices to new vertex IDs.
Thread safety | Unsafe |
---|
History 2
- 644 Change Tags of SetFaceVertices from [] to [CustomLuaState]
- 640 Add SetFaceVertices
SetFacsBonePose
Parameters (3) | ||
---|---|---|
action | FacsActionUnit | |
boneId | int64 | |
cframe | CFrame | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetFacsBonePose
SetFacsCorrectivePose
Parameters (3) | ||
---|---|---|
actions | Array | |
boneIds | Array | |
cframes | Array | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
SetFacsPose
Parameters (3) | ||
---|---|---|
action | FacsActionUnit | |
boneIds | Array | |
cframes | Array | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetFacsPose
SetNormal
Parameters (2) | ||
---|---|---|
normalId | int64 | |
normal | Vector3 | |
Returns (1) | ||
null |
Set the normal for a normal ID. This will change the normal value for every face vertex which is using the normal ID.
Thread safety | Unsafe |
---|
SetPosition
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
p | Vector3 | |
Returns (1) | ||
null |
Sets a vertex position in the mesh's local object space.
Thread safety | Unsafe |
---|
History 4
- 604 Change Parameters of SetPosition from (vertexId: int, p: Vector3) to (vertexId: int64, p: Vector3)
- 603 Change Parameters of SetPosition from (vertexId: int64, p: Vector3) to (vertexId: int, p: Vector3)
- 604 Change Parameters of SetPosition from (vertexId: int, p: Vector3) to (vertexId: int64, p: Vector3)
- 602 Add SetPosition
SetUV
Parameters (2) | ||
---|---|---|
uvId | int64 | |
uv | Vector2 | |
Returns (1) | ||
null |
Sets UV coordinates for a UV ID.
Thread safety | Unsafe |
---|
History 5
- 640 Change Parameters of SetUV from (vertexId: int64, uv: Vector2) to (uvId: int64, uv: Vector2)
- 604 Change Parameters of SetUV from (vertexId: int, uv: Vector2) to (vertexId: int64, uv: Vector2)
- 603 Change Parameters of SetUV from (vertexId: int64, uv: Vector2) to (vertexId: int, uv: Vector2)
- 604 Change Parameters of SetUV from (vertexId: int, uv: Vector2) to (vertexId: int64, uv: Vector2)
- 602 Add SetUV
SetVertexBoneWeights
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
boneWeights | Array | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
SetVertexBones
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
boneIDs | Array | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 670 Add SetVertexBones
SetVertexFaceColor
Parameters (3) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
colorId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
SetVertexFaceNormal
Parameters (3) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
normalId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
SetVertexFaceUV
Parameters (3) | ||
---|---|---|
vertexId | int64 | |
faceId | int64 | |
uvId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 1
- 667 Add SetVertexFaceUV
SkinningEnabled
Type | Default | |
---|---|---|
bool | __api_dump_failed_to_create_class__ |
Thread safety | ReadSafe |
---|---|
Category | Data |
Loaded/Saved | true |
History 3
- 672 Change Tags of SkinningEnabled from [] to [Deprecated]
- 648 Change Default of SkinningEnabled from false to __api_dump_failed_to_create_class__
- 624 Add SkinningEnabled
Triangulate
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
null |
Splits all faces on the mesh to be triangles. Currently this does nothing since only triangles can be created, but if your code relies on triangles, it's recommended that you call this method after calling AssetService:CreateEditableMeshAsync().
Thread safety | Unsafe |
---|
History 1
- 640 Add Triangulate
Removed members 15
CreateMeshPartAsync
Parameters (2) | Default | |
---|---|---|
initialSize | Vector3 | |
options | Dictionary | nil |
Returns (1) | ||
MeshPart |
Thread safety | Unsafe |
---|
History 6
- 660 Remove CreateMeshPartAsync
- 649 Change Tags of CreateMeshPartAsync from [Yields] to [Yields, Deprecated]
- 649 Change PreferredDescriptor of CreateMeshPartAsync from to CreateMeshPartAsync
- 648 Change Parameters of CreateMeshPartAsync from (options: Dictionary = nil) to (initialSize: Vector3, options: Dictionary = nil)
- 619 Change Parameters of CreateMeshPartAsync from (collisionFidelity: CollisionFidelity) to (options: Dictionary = nil)
- 602 Add CreateMeshPartAsync
GetAdjacentTriangles
Parameters (1) | ||
---|---|---|
triangleId | int64 | |
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 6
- 648 Remove GetAdjacentTriangles
- 640 Change Tags of GetAdjacentTriangles from [] to [Deprecated]
- 604 Change Parameters of GetAdjacentTriangles from (triangleId: int) to (triangleId: int64)
- 603 Change Parameters of GetAdjacentTriangles from (triangleId: int64) to (triangleId: int)
- 604 Change Parameters of GetAdjacentTriangles from (triangleId: int) to (triangleId: int64)
- 602 Add GetAdjacentTriangles
GetContent
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Content |
Thread safety | Unsafe |
---|
History 2
- 649 Remove GetContent
- 648 Add GetContent
GetTriangleVertices
Parameters (1) | ||
---|---|---|
triangleId | int64 | |
Returns (1) | ||
Tuple |
Thread safety | Unsafe |
---|
History 6
- 648 Remove GetTriangleVertices
- 640 Change Tags of GetTriangleVertices from [] to [Deprecated]
- 604 Change Parameters of GetTriangleVertices from (triangleId: int) to (triangleId: int64)
- 603 Change Parameters of GetTriangleVertices from (triangleId: int64) to (triangleId: int)
- 604 Change Parameters of GetTriangleVertices from (triangleId: int) to (triangleId: int64)
- 602 Add GetTriangleVertices
GetTriangles
Parameters (0) | ||
---|---|---|
No parameters. | ||
Returns (1) | ||
Array |
Thread safety | Unsafe |
---|
History 3
- 648 Remove GetTriangles
- 640 Change Tags of GetTriangles from [] to [Deprecated]
- 602 Add GetTriangles
GetVertexColor
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Color3 |
Thread safety | Unsafe |
---|
History 6
- 648 Remove GetVertexColor
- 640 Change Tags of GetVertexColor from [] to [Deprecated]
- 604 Change Parameters of GetVertexColor from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetVertexColor from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetVertexColor from (vertexId: int) to (vertexId: int64)
- 602 Add GetVertexColor
GetVertexColorAlpha
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
float |
Thread safety | Unsafe |
---|
History 6
- 648 Remove GetVertexColorAlpha
- 640 Change Tags of GetVertexColorAlpha from [] to [Deprecated]
- 604 Change Parameters of GetVertexColorAlpha from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetVertexColorAlpha from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetVertexColorAlpha from (vertexId: int) to (vertexId: int64)
- 602 Add GetVertexColorAlpha
GetVertexNormal
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
Vector3 |
Thread safety | Unsafe |
---|
History 6
- 648 Remove GetVertexNormal
- 640 Change Tags of GetVertexNormal from [] to [Deprecated]
- 604 Change Parameters of GetVertexNormal from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of GetVertexNormal from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of GetVertexNormal from (vertexId: int) to (vertexId: int64)
- 602 Add GetVertexNormal
Raycast
Parameters (2) | ||
---|---|---|
origin | Vector3 | |
direction | Vector3 | |
Returns (1) | ||
Tuple |
Thread safety | Unsafe |
---|
RemoveTriangle
Parameters (1) | ||
---|---|---|
triangleId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 6
- 648 Remove RemoveTriangle
- 640 Change Tags of RemoveTriangle from [] to [Deprecated]
- 604 Change Parameters of RemoveTriangle from (triangleId: int) to (triangleId: int64)
- 603 Change Parameters of RemoveTriangle from (triangleId: int64) to (triangleId: int)
- 604 Change Parameters of RemoveTriangle from (triangleId: int) to (triangleId: int64)
- 602 Add RemoveTriangle
RemoveVertex
Parameters (1) | ||
---|---|---|
vertexId | int64 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 6
- 648 Remove RemoveVertex
- 640 Change Tags of RemoveVertex from [] to [Deprecated]
- 604 Change Parameters of RemoveVertex from (vertexId: int) to (vertexId: int64)
- 603 Change Parameters of RemoveVertex from (vertexId: int64) to (vertexId: int)
- 604 Change Parameters of RemoveVertex from (vertexId: int) to (vertexId: int64)
- 602 Add RemoveVertex
SetVertexColor
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
color | Color3 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 6
- 648 Remove SetVertexColor
- 640 Change Tags of SetVertexColor from [] to [Deprecated]
- 604 Change Parameters of SetVertexColor from (vertexId: int, color: Color3) to (vertexId: int64, color: Color3)
- 603 Change Parameters of SetVertexColor from (vertexId: int64, color: Color3) to (vertexId: int, color: Color3)
- 604 Change Parameters of SetVertexColor from (vertexId: int, color: Color3) to (vertexId: int64, color: Color3)
- 602 Add SetVertexColor
SetVertexColorAlpha
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
alpha | float | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 6
- 648 Remove SetVertexColorAlpha
- 640 Change Tags of SetVertexColorAlpha from [] to [Deprecated]
- 604 Change Parameters of SetVertexColorAlpha from (vertexId: int, alpha: float) to (vertexId: int64, alpha: float)
- 603 Change Parameters of SetVertexColorAlpha from (vertexId: int64, alpha: float) to (vertexId: int, alpha: float)
- 604 Change Parameters of SetVertexColorAlpha from (vertexId: int, alpha: float) to (vertexId: int64, alpha: float)
- 602 Add SetVertexColorAlpha
SetVertexNormal
Parameters (2) | ||
---|---|---|
vertexId | int64 | |
vnormal | Vector3 | |
Returns (1) | ||
null |
Thread safety | Unsafe |
---|
History 6
- 648 Remove SetVertexNormal
- 640 Change Tags of SetVertexNormal from [] to [Deprecated]
- 604 Change Parameters of SetVertexNormal from (vertexId: int, vnormal: Vector3) to (vertexId: int64, vnormal: Vector3)
- 603 Change Parameters of SetVertexNormal from (vertexId: int64, vnormal: Vector3) to (vertexId: int, vnormal: Vector3)
- 604 Change Parameters of SetVertexNormal from (vertexId: int, vnormal: Vector3) to (vertexId: int64, vnormal: Vector3)
- 602 Add SetVertexNormal